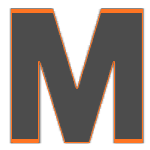
生产者和消费者指的是两个不同的线程类对象,操作同一资源的情况。
结构图:
package javatest.pattern;
import java.util.LinkedList;
import java.util.Random;
/**
* @description: 生产者消费者设计模式
* @modifyContent:
* @author: Maple Chan
* @date: 2020-07-29 20:31:06
* @version: 0.0.1
*/
public class ProducerAndConsumer {
public static void main(String[] args) {
new Producer().start();
new ConsumerA().start();
new ConsumerB().start();
}
}
class Producer extends Thread {
private void produce() {
Goods store = Goods.getInstance();
// do produce
long product = new Random().nextLong();
// put into store
synchronized (store) {
System.out.println("生产产品:" + product);
Goods.goodList.add(product);
}
}
@Override
public void run() {
int count = 3;
while (true) {
long sleepTime = new Random().nextInt(10) * 100;
try {
Thread.sleep(sleepTime);
} catch (Exception e) {
// TODO: handle exception
}
this.produce();
if (count-- < 0) {
break;
}
}
return;
}
}
class ConsumerA extends Thread {
private void consumer() {
Goods store = Goods.getInstance();
synchronized (store) {
Long product = Goods.goodList.getFirst();
Goods.goodList.remove(product);
System.out.println("消费产品:" + product);
}
}
@Override
public void run() {
while (true) {
long sleepTime = new Random().nextInt(10) * 100;
try {
Thread.sleep(sleepTime);
} catch (Exception e) {
// TODO: handle exception
}
if (Goods.goodList.size() > 0) {
this.consumer();
}
}
}
}
class ConsumerB extends Thread {
private void consumer() {
Goods store = Goods.getInstance();
synchronized (store) {
Long product = Goods.goodList.getFirst();
Goods.goodList.remove(product);
System.out.println("消费产品:" + product);
}
}
@Override
public void run() {
while (true) {
long sleepTime = new Random().nextInt(10) * 120;
try {
Thread.sleep(sleepTime);
} catch (Exception e) {
// TODO: handle exception
}
// 库存有东西才会进行消费
if (Goods.goodList.size() > 0) {
this.consumer();
}
}
}
}
class Goods {
public static LinkedList<Long> goodList;
private static Goods singletonGoods;
private Goods() {
}
static {
goodList = new LinkedList<>();
singletonGoods = new Goods();
}
/**
* 单例
*/
public static Goods getInstance() {
return singletonGoods;
}
}
输出如下所示:
/*
生产产品:6805117464760743258
消费产品:6805117464760743258
生产产品:1599562522443449507
生产产品:1041166688112309013
消费产品:1599562522443449507
生产产品:124370676431480001
消费产品:1041166688112309013
生产产品:7414022159762949506
消费产品:124370676431480001
消费产品:7414022159762949506
代码中生产5个数据,之后消费将一直等待。
*/